PHP
<form enctype="multipart/form-data" action="__URL__" method="POST">
<!-- MAX_FILE_SIZE は、必ず "file" input フィールドより前に、valueは単位:バイトでサイズ制限 -->
<input type="hidden" name="MAX_FILE_SIZE" value="30000" />
<!-- input 要素の name 属性の値が、$_FILES 配列のキーになる -->
<input name="userfile" type="file" />
<input type="submit" value="ファイルを送信" />
</form>
$_FILESの配列
PHP
move_uploaded_file //サーバーに一時保存されたファイルをmove_uploaded_fileで指定場所に移動
PHP
//サーバーに一時保存されたファイル情報は配列形式
//$_FILES[inputのname][アップロードされたファイルの情報]
$_FILES[inputのname]['name'] //元のファイル名
$_FILES[inputのname]['tmp_name'] //サーバーに一時保存されたファイル名
$_FILES[inputのname]['error'] //エラー内容
$_FILES[inputのname]['type'] //ファイルタイプ
$_FILES[inputのname]['size'] //ファイルサイズ
配列キー | 一時保存された内容 |
---|---|
name | 元のファイル名 |
tmp_name | サーバーに一時保存されたファイル名 |
error | エラー内容 |
type | ファイルタイプ |
size | ファイルサイズ |
エラー: $_FILES[”][‘error’]
[‘error’]は0~8の数字で返される。エラーがない場合は”0” $_FILES[”][‘error’]に値5が無いので注意。コード | エラー定数 | 説明 |
---|---|---|
0 | UPLOAD_ERR_OK | エラーなし |
1 | UPLOAD_ERR_INI_SIZE | php.iniのupload_max_filesizeのサイズを超えている |
2 | UPLOAD_ERR_FORM_SIZE | HTMLフォームで指定された MAX_FILE_SIZE を超えている |
3 | UPLOAD_ERR_PARTIAL | 一部のみしかアップロードされていない |
4 | UPLOAD_ERR_NO_FILE | アップロードされなかった |
6 | UPLOAD_ERR_NO_TMP_DIR | サーバー一時保管フォルダがない |
7 | UPLOAD_ERR_CANT_WRITE | ディスクへの書き込みに失敗 |
8 | UPLOAD_ERR_EXTENSION | PHPの拡張モジュールがアップロードを中止した |
POST遷移かの判定
PHP
//POSTリクエストによるページ遷移かチェック
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
//適正なPOSTによる取得
}else{
//POSTリクエストによる遷移じゃない場合
echo '不正なアクセスです!';
}//
ファイル形式のチェック
PHP
//jpeg形式じゃない場合
if ($_FILES['upimg']['type'] !=='image/jpeg'){
echo 'JPEG形式の画像を選択してください!';
}//
// PNG形式じゃない場合
if($_FILES['upimg']['type'] !=='image/png'){
echo 'PNG形式の画像を選択してください!';
}//
//テキストファイルじゃない場合
if ($_FILES['upload']['type'] !== 'text/plain') {
echo 'テキストファイルを選択してください!';
}//
エラー無しなら保存する
PHP
$uploadfile ="/files/memo.txt";//ファイルの保存先
//エラー無し
if($_FILES['upload']['error'] === 0) {
// アップロードされたファイルに、パスとファイル名を設定して保存
move_uploaded_file($_FILES['upload']['tmp_name'], $uploadfile);
// 完了メッセージを表示
echo '完了!';
}//
画像をアップロード、リサイズする
PHP
<?php
//フォーム返値を判別--------
if($_FILES['upimg']['size'] < 0){
echo "返り値がありません。";
}else{
echo ($_FILES['upimg']['type'])."<br>";
//画像拡張子チェック----
if( $_FILES['upimg']['type'] =='image/jpeg' || $_FILES['upimg']['type'] =='image/png' ){
date_default_timezone_set('Asia/Tokyo');//タイムエリア
$set_date = date('Ymd_His');//日付
$img_name = $set_date. "_" .$_FILES['upimg']['name']; //変更後ファイル名
$setimg_area = "img/".$img_name ; // (保存先ファイル名)ファイル名_$date
move_uploaded_file( $_FILES['upimg']['tmp_name'] , $setimg_area );
//サムネthumb作成------
$moto_img = imagecreatefromjpeg("img/$img_name");
list($w,$h) = getimagesize("img/$img_name");
$new_h = 100;
$new_w = $w *100 /$h;
$mythumb = imagecreatetruecolor($new_w, $new_h );
imagecopyresized($mythumb , $moto_img , 0,0,0,0 , $new_w, $new_h,$w, $h);
imagejpeg($mythumb , "thumb/$img_name");
//サムネ表示--
echo "サムネUP成功";
?>
<img src="thumb/<?php echo $img_name ?>" >
<?php
}else{
echo"不適切な画像です";
}//end_if
}//end_if
?>
ディレクトリ内ファイル表示
保存した画像ファイルの一覧を表示する。dir関数を利用してファイル一覧を表示するPHP
$dir = dir("img/");
while(FALSE !== ($fileName = $dir->read())){
if ($fileName != "." && $fileName != "..") {
echo $fileName ."<br>";
}
}
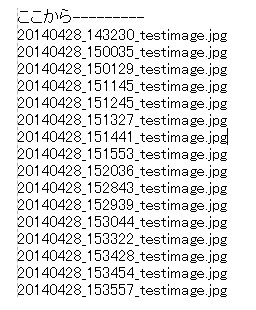
画像読込、アップロードとCookieで画像name保存のサンプル
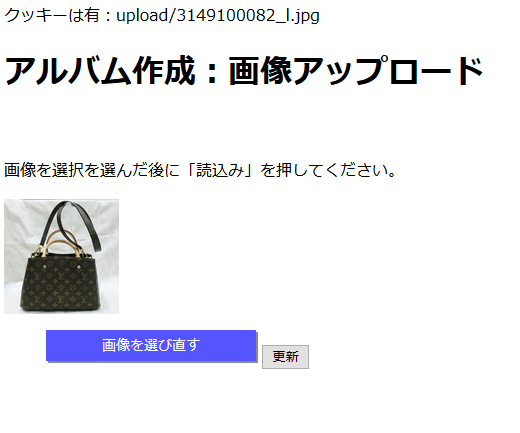
処理内容
画像判定、画像保存、クッキー保存、inputボタンの書き換え、クッキー保存画像名で表示 ・ファイルがjpgかpngなら画像をupload/フォルダに保存 ・「upload/画像名」をCookiに保存する ・Cookiが保存されていたら画像を表示して更新ボタンを表示する(PHP内容)upload-top.php
PHP
<?php
//画像01---
//画像01の表示
$file01_mes = "";
$file01_url = "img/no_photo.png";
$file01_button = "読込み";
if ($_FILES['upfile'] <>"" ){ //POSTファイルのがあったら
if(isset($_FILES)&& isset($_FILES['upfile']) && is_uploaded_file($_FILES['upfile']['tmp_name'])){
if(!file_exists('upload')){
mkdir('upload');
}
//画像の確認
if(
( $_FILES['upfile'] > 0 ) &&
( $_FILES['upfile']['type'] =='image/jpeg' || $_FILES['upfile']['type'] =='image/png' ) &&
( ( strtolower (mb_strrchr($_FILES['upfile']['name'],'.',FALSE )) == ".jpg" ) ||
( strtolower (mb_strrchr($_FILES['upfile']['name'],'.',FALSE )) == ".png" )
)
){
$a = 'upload/' . basename($_FILES['upfile']['name']);
if(move_uploaded_file($_FILES['upfile']['tmp_name'], $a)){
$msg = $a. 'のアップロードに成功しました';
//Cookiに画像名を保存 time()1時間
setcookie('photo_up01', $a, time()+ 60 * 60 * 1 );
echo "Cooki保存";
$file01_mes = "画像を変更する";
$file01_url = $a;
$file01_button = "更新";
}else {
$msg = 'アップロードに失敗しました';
}//end_if
}else{
$msg = 'jpgかpng形式の画像ファイルを選択してください';
if( $_COOKIE['photo_up01'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選び直す";
$file01_url = $_COOKIE['photo_up01'] ;
$file01_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選択してください";
$file01_url = "img/no_photo.png";
$file01_button = "読込み";
}//end_if
}//end_if
}//end_if
}else{ //POSTファイルが無かったら
if( $_COOKIE['photo_up01'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選び直す";
$file01_url = $_COOKIE['photo_up01'] ;
$file01_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選択してください";
$file01_url = "img/no_photo.png";
$file01_button = "読込み";
}//end_if
}//end_if
?>
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
</head>
<body>
<h1>アルバム作成:画像アップロード</h1>
<?php
//メッセージ作成
if(isset($msg) && $msg == true){
echo '<p>'. $msg . '</p>';
}
?>
<br>
<p>画像を選択を選んだ後に「読込み」を押してください。</p>
<p> <img src="<?php echo ($file01_url); ?>" alt="登録済画像01" width="10%" />
<form action="upload-top.php" method="post" enctype="multipart/form-data">
<label class="my-file-input"><input type="file" id="test3" name="upfile" /><?php echo ($file01_mes); ?></label>
<input type="submit" value="<?php echo ($file01_button); ?>">
</form>
</p>
</body>
<!-- Labeの変更 -->
<style type="text/css">
<!--
label {
margin-left: 3em;
}
.my-file-input {
display: inline-block;
padding: 5px;
width: 200px;
text-align: center;
white-space: nowrap;
overflow: hidden;
font-size: 14px;
text-overflow: ellipsis;
background-color: #55f;
color: white;
box-shadow: #888 2px 2px 1px;
cursor: pointer;
}
.my-file-input:hover {
background-color: #88f;
}
.my-file-input:hover {
background-color: #88f;
}
.my-file-input:active {
box-shadow: #888 1px 1px 1px;
position: relative;
top: 1px; left: 1px;
}
.my-file-input input {
display: none;
}
</style>
<script>
document.getElementById("test2").addEventListener("change", function(e){
e.target.nextSibling.nodeValue = e.target.files.length ? e.target.files[0].name : "ファイルを選ぶぜ!";
});
document.getElementById("test3").addEventListener("change", function(e){
e.target.nextSibling.nodeValue = e.target.files.length ? e.target.files[0].name : "ファイルを選ぶぜ!";
});
</script>
</html>
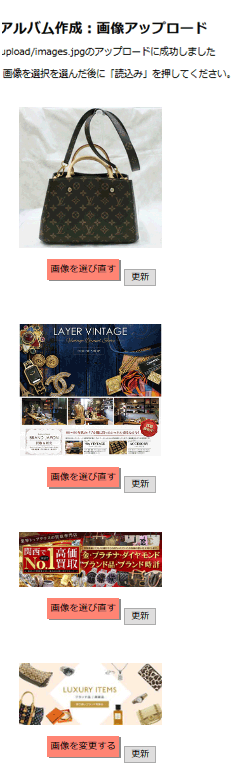
4つの画像をアップロード
重複は登録せず、画像を選びなおすときは古い画像を削除して更新するPHP
<?php
//画像01---
//画像01の表示
$file01_mes = "";
$file01_url = "img/no_photo.png";
$file01_button = "読込み";
echo "<br>01:".$_COOKIE['photo_up01'];
echo "<br>02:".$_COOKIE['photo_up02'];
echo "<br>03:".$_COOKIE['photo_up03'];
echo "<br>04:".$_COOKIE['photo_up04'];
echo"<br><br>";
//Cooki画像のセット‐------------------------
if( $_COOKIE['photo_up01'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選び直す";
$file01_url = $_COOKIE['photo_up01'] ;
$file01_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up01']."<br>";
$file01_mes = "画像を選択してください";
$file01_url = "img/no_photo.png";
$file01_button = "読込み";
}//end_if
//Cooki画像のセット‐------------------------
if( $_COOKIE['photo_up02'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up02']."<br>";
$file02_mes = "画像を選び直す";
$file02_url = $_COOKIE['photo_up02'] ;
$file02_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up02']."<br>";
$file02_mes = "画像を選択してください";
$file02_url = "img/no_photo.png";
$file02_button = "読込み";
}//end_if
//Cooki画像のセット‐------------------------
if( $_COOKIE['photo_up03'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up03']."<br>";
$file03_mes = "画像を選び直す";
$file03_url = $_COOKIE['photo_up03'] ;
$file03_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up03']."<br>";
$file03_mes = "画像を選択してください";
$file03_url = "img/no_photo.png";
$file03_button = "読込み";
}//end_if
//Cooki画像のセット‐------------------------
if( $_COOKIE['photo_up04'] != ""){ //Cooliがあったら
echo "クッキーは有:".$_COOKIE['photo_up04']."<br>";
$file04_mes = "画像を選び直す";
$file04_url = $_COOKIE['photo_up04'] ;
$file04_button = "更新";
}else{
echo "クッキー無し".$_COOKIE['photo_up04']."<br>";
$file04_mes = "画像を選択してください";
$file04_url = "img/no_photo.png";
$file04_button = "読込み";
}//end_if
//------------------------------------
if ($_FILES['upfile'] <>"" ){ //POSTファイルがあったら
if(isset($_FILES)&& isset($_FILES['upfile']) && is_uploaded_file($_FILES['upfile']['tmp_name'])){
if(!file_exists('upload')){
mkdir('upload');
}
//画像の確認
if(
( $_FILES['upfile'] > 0 ) &&
( $_FILES['upfile']['type'] =='image/jpeg' || $_FILES['upfile']['type'] =='image/png' ) &&
( ( strtolower (mb_strrchr($_FILES['upfile']['name'],'.',FALSE )) == ".jpg" ) ||
( strtolower (mb_strrchr($_FILES['upfile']['name'],'.',FALSE )) == ".png" )
)
){
$a = 'upload/' . basename($_FILES['upfile']['name']);//画像のURL作成
$cookiname = "photo_up". $_POST['cooki_sw'];//Cooki名の作成
//Cookiに保存されていないかの確認
if( ($_COOKIE['photo_up01'] == $a ) || ($_COOKIE['photo_up02'] == $a ) ||
($_COOKIE['photo_up03'] == $a ) || ($_COOKIE['photo_up03'] == $a )
){
$msg = $a. 'の画像は既に選択されています';
}else{
//画像を保存する------
if(move_uploaded_file($_FILES['upfile']['tmp_name'], $a)){ //画像が保存できたら
$msg = $a. 'のアップロードに成功しました';
if ( $_COOKIE["$cookiname"]!= ""){
$dalete_img = $_COOKIE["$cookiname"];
echo $dalete_img."ファイルを削除します";
unlink("$dalete_img"); //古い画像の削除
}
setcookie($cookiname , $a, time()+ 60 * 60 * 1 ); //Cookiに画像名を保存 time()1時間
echo $_POST['cooki_sw']."**Cooki保存";
if($_POST['cooki_sw'] == 01){
$file01_mes = "画像を変更する";
$file01_url = $a;
$file01_button = "更新";
}
if($_POST['cooki_sw'] == 02){
$file02_mes = "画像を変更する";
$file02_url = $a;
$file02_button = "更新";
}
if($_POST['cooki_sw'] == 03){
$file03_mes = "画像を変更する";
$file03_url = $a;
$file03_button = "更新";
}
if($_POST['cooki_sw'] == 04){
$file04_mes = "画像を変更する";
$file04_url = $a;
$file04_button = "更新";
}
}else {//アップロードに失敗したら
$msg = 'アップロードに失敗しました';
}//end_if
//画像保存するここまで----
}//end_if
}else{
$msg = 'jpgかpng形式の画像ファイルを選択してください';
}//end_if
}//end_if
}//end_if
?>
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
</head>
<body>
<h1>アルバム作成:画像アップロード</h1>
<?php
//メッセージ作成
if(isset($msg) && $msg == true){
echo '<p>'. $msg . '</p>';
}
?>
<br>
<p>画像を選択を選んだ後に「読込み」を押してください。</p>
<p> <img src="<?php echo ($file01_url); ?>" alt="登録済画像01" width="10%" />
<form action="upload-top.php" method="post" enctype="multipart/form-data">
<label class="my-file-input"><input type="file" id="test3" name="upfile" /><?php echo ($file01_mes); ?></label>
<input type="hidden" name="cooki_sw" value="01">
<input type="submit" value="<?php echo ($file01_button); ?>">
</form>
</p>
<br>
<p> <img src="<?php echo ($file02_url); ?>" alt="登録済画像02" width="10%" />
<form action="https://www.brandshop.co.jp/brand-kaitori/form/upload-top.php" method="post" enctype="multipart/form-data">
<label class="my-file-input"><input type="file" id="test3" name="upfile" /><?php echo ($file02_mes); ?></label>
<input type="hidden" name="cooki_sw" value="02">
<input type="submit" value="<?php echo ($file02_button); ?>">
</form>
</p>
<br>
<p> <img src="<?php echo ($file03_url); ?>" alt="登録済画像03" width="10%" />
<form action="https://www.brandshop.co.jp/brand-kaitori/form/upload-top.php" method="post" enctype="multipart/form-data">
<label class="my-file-input"><input type="file" id="test3" name="upfile" /><?php echo ($file03_mes); ?></label>
<input type="hidden" name="cooki_sw" value="03">
<input type="submit" value="<?php echo ($file03_button); ?>">
</form>
</p>
<br>
<p> <img src="<?php echo ($file04_url); ?>" alt="登録済画像04" width="10%" />
<form action="https://www.brandshop.co.jp/brand-kaitori/form/upload-top.php" method="post" enctype="multipart/form-data">
<label class="my-file-input"><input type="file" id="test3" name="upfile" /><?php echo ($file04_mes); ?></label>
<input type="hidden" name="cooki_sw" value="04">
<input type="submit" value="<?php echo ($file04_button); ?>">
</form>
</p>
<br>
</body>
<!-- Labeの変更 -->
<style type="text/css">
<!--
label {
margin-left: 3em;
}
.my-file-input {
display: inline-block;
padding: 5px;
width: auto;
text-align: center;
white-space: nowrap;
overflow: hidden;
font-size: 14px;
text-overflow: ellipsis;
background-color: #fa8072;
color: #000000;
box-shadow: #888 2px 2px 1px;
cursor: pointer;
}
.my-file-input:hover {
background-color: #cd5c5c;
}
.my-file-input:active {
box-shadow: #888 1px 1px 1px;
position: relative;
top: 1px; left: 1px;
}
.my-file-input input {
display: none;
}
</style>
<script>
document.getElementById("test2").addEventListener("change", function(e){
e.target.nextSibling.nodeValue = e.target.files.length ? e.target.files[0].name : "ファイルを選ぶぜ!";
});
document.getElementById("test3").addEventListener("change", function(e){
e.target.nextSibling.nodeValue = e.target.files.length ? e.target.files[0].name : "ファイルを選ぶぜ!";
});
</script>
</html>